Canvas 图形变换
一、Canvas 平移
cxt.translate(x, y)
用于平移图形。
参数:
x
:水平偏移量;y
:垂直偏移量。
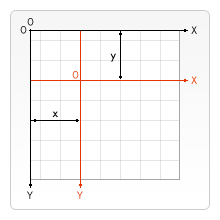
示例:
ctx.translate(10, 10);
ctx.fillRect(0, 0, 100, 100);
效果如下:
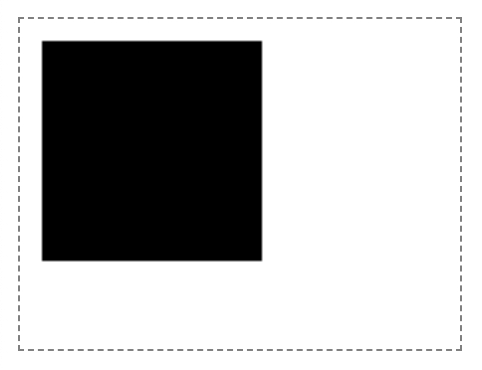
二、Canvas 旋转
cxt.rotate(angle)
用于旋转图形,计算方式:degree * Math.PI / 180
。
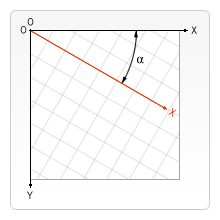
示例:
ctx.rotate(10 * Math.PI / 180);
ctx.fillRect(30, 30, 60, 60);
效果如下:
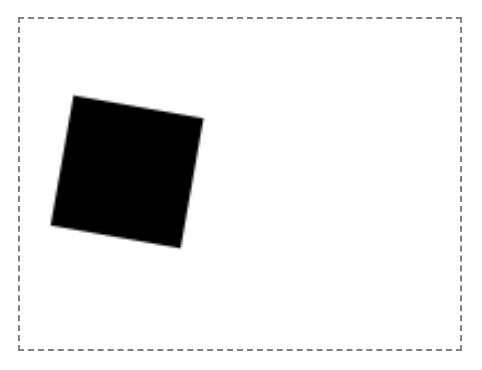
旋转中心是 Canvas 的坐标圆点。如果想改变中心点,我们可以通过 translate()
方法移动 Canvas,再进行旋转。
三、Canvas 缩放〡翻转
ctx.scale(x, y)
用于缩放或翻转图形。
1、缩放
x、y 默认为 1(原始大小),小于 1 缩小,大于 1 放大;
示例:
// 缩放前
ctx.beginPath()
ctx.fillStyle = 'pink'
ctx.fillRect(30, 30, 60, 60)
// 缩放后
ctx.beginPath()
ctx.scale(2, 0.5)
ctx.fillStyle = 'aquamarine'
ctx.fillRect(30, 30, 60, 60)
效果如下:
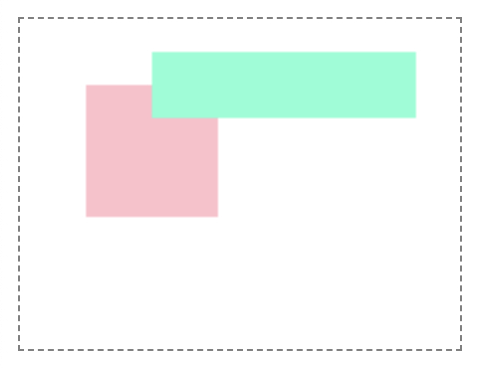
注意缩放时图形坐标也会跟着变化。
2、翻转
ctx.scale(-1, 1)
:水平翻转;ctx.scale(1, -1)
:垂直翻转。
示例:
// 翻转前
ctx.beginPath()
ctx.font = "bold 48px serif"
ctx.fillText('Hello!', 0, 60)
// 翻转后
ctx.beginPath()
ctx.scale(-1, 1)
ctx.font = "bold 48px serif"
ctx.fillText('Hello!', -200, 120)
效果如下:
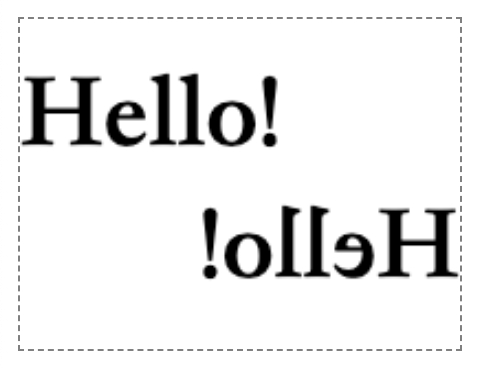
四、Canvas 变形矩阵
ctx.transform(a, b, c, d, e, f)
使用矩阵多次叠加当前变换的方法,用于缩放、旋转、移动和倾斜图形。

参数:
a
:水平方向的缩放b
:竖直方向的倾斜偏移c
:水平方向的倾斜偏移d
:竖直方向的缩放e
:水平方向的移动f
:竖直方向的移动
setTransform(a, b, c, d, e, f)
用于将当前的变形矩阵重置为单位矩阵,然后用相同的参数调用 transform 方法;
resetTransform()
用于重置当前变形为单位矩阵,等同于 ctx.setTransform(1, 0, 0, 1, 0, 0)
示例:
// 变形前
ctx.beginPath()
ctx.fillStyle = 'pink'
ctx.fillRect(20, 20, 50, 50);
// 变形后
ctx.beginPath()
ctx.transform(1, 1, 0, 1, 0, 0);
ctx.fillStyle = 'aquamarine'
ctx.fillRect(20, 20, 50, 50);
效果如下:
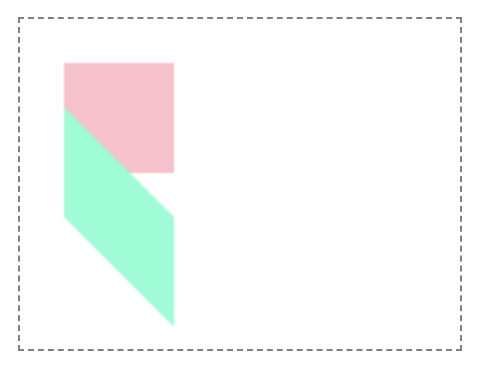
五、Canvas 裁剪
clip()
用于从原始画布中剪切任意形状和尺寸。语法如下:
ctx.clip()
ctx.clip(fillRule)
ctx.clip(path, fillRule)
参数:
fillRule
:判断一个点是在路径内还是在路径外。可选值:nonzero(默认)
:非零环绕原则;evenodd
:奇偶环绕原则;
path
:需要剪切的 Path2D 路径。
示例:
// 未裁剪
ctx.beginPath()
ctx.arc(100, 100, 75, 0, Math.PI * 2, false);
ctx.fillStyle = 'pink'
ctx.fillRect(0, 0, 100, 100);
// 已裁剪
ctx.beginPath()
ctx.arc(100, 100, 75, 0, Math.PI * 2, false);
ctx.clip();
ctx.fillStyle = 'aquamarine'
ctx.fillRect(100, 0, 200, 100);
效果如下:
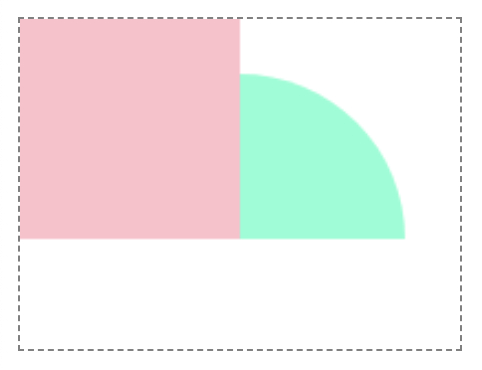